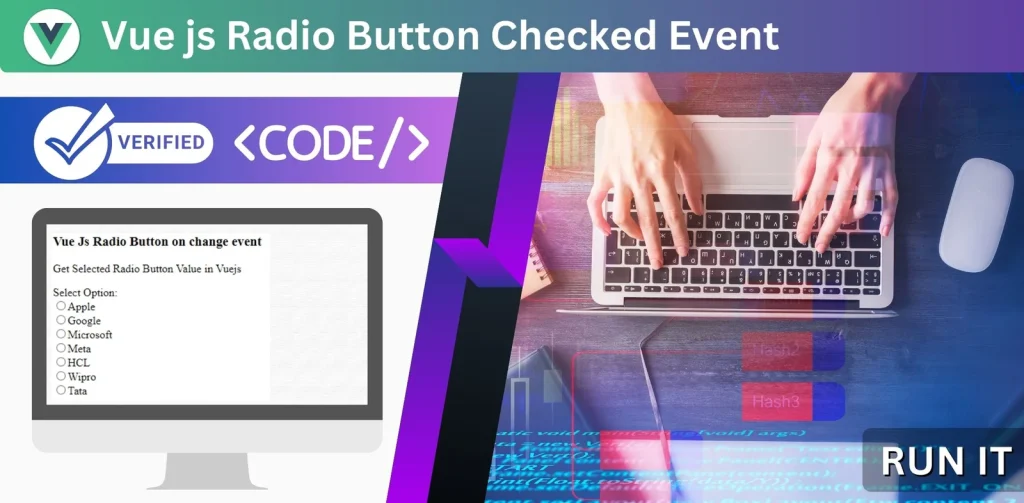
Vue js radio button checked event/radio on change event: In Vue.js, the “change” event is emitted by a radio input when its value is changed by user interaction. To handle this event, you can use the v-on directive to bind a method to the change event.
A radio button can be bound to a variable, and its checked state can be controlled by that variable’s value. When the radio button is clicked, the value of the variable can be updated, and this can trigger an event. This event can be used to perform some action, such as updating other parts of the component or making an API call. In this example, we will learn how to get the checked radio button value on an onchange event.
How to get the radio button value on a change event?
In Vue.js, you can handle a radio button being checked by using the “v-on” directive to listen for a “change” event on the radio button element. You can then use a method in your Vue instance to handle the event and perform any necessary actions. Here’s an example of how you might do this:
Vue Js radio button click event | Example
<div id="app">
<div v-for='(option,index) in options'>
<input type="radio" @change="changeEvent" :id="index" v-model="selectedValue" :value="option">{{option}}
</div>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
options: ['Apple', 'Google', 'Microsoft', 'Meta', 'HCL', 'Wipro', 'Tata'],
selectedValue: '',
}
},
methods: {
changeEvent(event) {
alert('Selected Value :' + event.target.value)
}
}
});
</script>
Output of above example
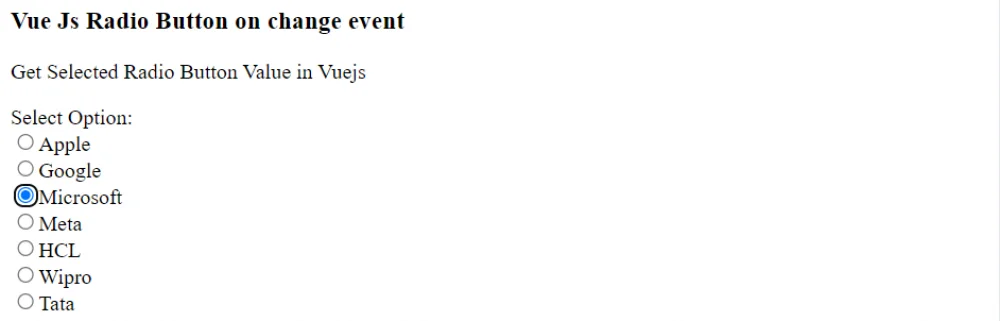